In this article, we’ll explore ten essential SQL commands that are commonly used in database management. SQL (Structured Query Language) is a powerful tool for querying and manipulating data in relational databases. Whether you’re a beginner or an experienced developer, these examples will help you understand the versatility and efficiency of SQL commands.
1. SELECT <a name=”select”></a>
The SELECT
statement is one of the fundamental SQL commands used to retrieve data from a database table. It allows you to specify the columns you want to retrieve and apply filtering conditions using the WHERE
clause.
Example:
sqlCopy codeSELECT first_name, last_name, age FROM employees WHERE department = 'HR';
2. INSERT <a name=”insert”></a>
The INSERT
statement is used to insert new records into a database table. You can provide specific values for each column or use a subquery to insert data from another table.
Example:
sqlCopy codeINSERT INTO products (product_name, price, quantity) VALUES ('Laptop', 1200, 50);
3. UPDATE <a name=”update”></a>
The UPDATE
statement allows you to modify existing records in a table. It is often used with the WHERE
clause to update specific rows based on certain conditions.
Example:
sqlCopy codeUPDATE customers SET city = 'New York' WHERE country = 'USA';
4. DELETE <a name=”delete”></a>
The DELETE
statement is used to remove one or more rows from a table. Like the UPDATE
statement, it can also be combined with the WHERE
clause to delete specific rows.
Example:
sqlCopy codeDELETE FROM orders WHERE order_date < '2023-01-01';
5. CREATE TABLE <a name=”create-table”></a>
The CREATE TABLE
statement allows you to create a new table with the specified columns and their data types. It’s a crucial command for defining the structure of your database.
Example:
sqlCopy codeCREATE TABLE employees (
emp_id INT PRIMARY KEY,
emp_name VARCHAR(50),
department VARCHAR(30),
salary DECIMAL(10, 2)
);
6. ALTER TABLE <a name=”alter-table”></a>
The ALTER TABLE
statement is used to modify an existing table. You can add, modify, or drop columns, as well as change the data type of a column.
Example:
sqlCopy codeALTER TABLE customers ADD COLUMN date_of_birth DATE;
7. DROP TABLE <a name=”drop-table”></a>
The DROP TABLE
statement is used to delete an entire table from the database. Be cautious when using this command, as it permanently removes all data in the table.
Example:
sqlCopy codeDROP TABLE products;
8. JOIN <a name=”join”></a>
The JOIN
operation allows you to combine rows from two or more tables based on a related column. It’s a powerful command for querying data from multiple tables simultaneously.
Example:
sqlCopy codeSELECT orders.order_id, customers.first_name, customers.last_name
FROM orders
JOIN customers ON orders.customer_id = customers.customer_id;
9. GROUP BY <a name=”group-by”></a>
The GROUP BY
statement is used to group rows with similar values in one or more columns. It’s commonly used with aggregate functions like COUNT
, SUM
, AVG
, etc.
Example:
sqlCopy codeSELECT department, COUNT(*) as num_employees
FROM employees
GROUP BY department;
10. ORDER BY <a name=”order-by”></a>
The ORDER BY
statement allows you to sort the result set based on one or more columns, either in ascending or descending order.
Example:
sqlCopy codeSELECT product_name, price
FROM products
ORDER BY price DESC;
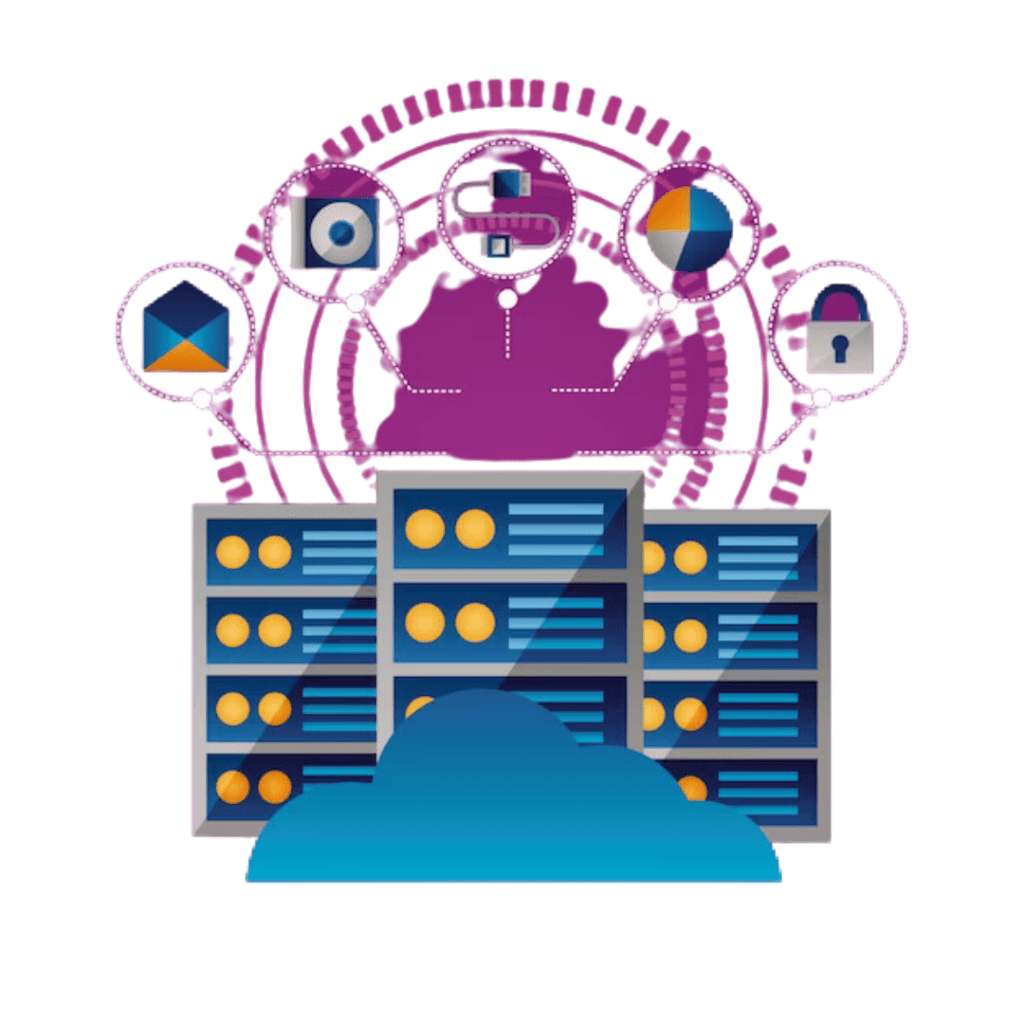
SQL commands are the building blocks of interacting with databases effectively. By mastering these ten examples, you’ll have a solid foundation for querying, updating, and managing data in your SQL databases. Practice and experimentation will further enhance your SQL skills, enabling you to tackle more complex database tasks with confidence. Happy coding! :ahmad: